Seventh Edition: Kip R. Irvine
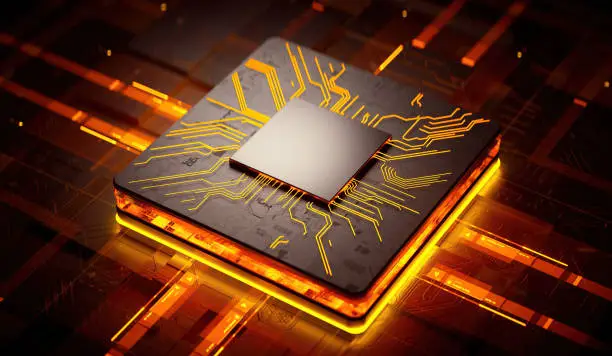
I started reading this book recently in hope of gaining a better understanding of operating systems. I think that the section reviews are great questions and here I will post up, section by section. 17 Chapters lets go!
Basic Concepts
Section 1.1.3 Review
- How do assemblers and linkers work together?
An assembler is a utility program that converts assembly source code into machine code. A linker is a utility program that combines files created by an assembler into a single executable file.
- How will studying assembly language enhance your understanding of operating systems?
Assembly language helps you understand the interaction between computer hardware, operating systems and application programs. How application programs communicate with the computer's operating system through interrupt handlers, system calls, and common memory areas. Assembly also helps learning how the operating system loads and executes application programs.
- What is meant by a one-to-many relationship when comparing a high-level language to machine language?
High level languages have a one-to-many relationship with machine code and assembly, meaning one statement in Java, Python... = many assembly/ machine language instuctions.
- Explain the concept of portability as it applies to programming languages.
A program who's source code can be compiled and ran on a wide variety of systems would be considered portable, like C or C++. Assembly language is not portable because, it is designed for a specific processor family.
- Is assembly language for x86 processors the same as the Vax or Motorola 68×00?
No, there are a number of different assembly languages based on a processor family.. Vax is one of its own and Motorola is also going to have its own specific assembly language.
- Give an example of an embedded systems application.
short programs stored in single purpose devices. Like A/C control systems, hard drives, modems, sound cards, video cards, telephones, automobile fuel and ignition systems, printers, security systems..
- What is a device driver?
A program that translates general operating system commands into specific references to hardware details.
- Do you suppose type checking on pointer variables is stronger in assembly or in c & C++?
Type checking on pointer variables is stricter in C & C++. C++ does not allow a pointer of one type to be assigned to a pointer of another type. Assembly language has no restrictions regarding pointers.
- Name two types of applications that would better suit assembly language.
Hardware device driver, embedded systems, video games.
- Why would a high-level language not usually be used when writing a program that directly accesses a printer port?
A high-level language might not provide direct access to the hardware, and even if it does, awkward coding techniques often will have to be used.
- Why is assembly language not usually used when writing large application programs?
Minimal form structure. Difficulties maintaining code.
- Challenge: Translate the following C++ into assembly. x = (y * 4) + 3;
mov eax,y; move 4 to EAX mov ebx,4; move 4 to EBX imul ebx; EAX = EAX * EBX add eax,3; move EAX to X
Section 1.2.1 Review
- In your own words, describe the virtual machine concept.
The virtual machine concept, is the relation of a computers hardware at a bottom level and High-level programming languages to assist in creating complex programs through the abstraction of layers. The translation of high-level languages using compilers into lower level languages to then be executed on a computers hardware.
- Why do you suppose translated programs often execute more quickly than interpreted ones?
A translated program can be directly executed while an interpreted one must be translated while it is running.
- (True/ False): When an interpreted program written in language L1 runs, each of its instructions is decoded and executed by a program written in language L0.
True
- Explain the importance of translation when dealing with languages at different virtual machine levels.
Translation is important with machines at higher levels because in order to communicate with the hardware at the lowest level, the high level code needs to be converted into executable programs the machine can understand.
- At which level does assembly language appear in the virtual machine example shown in this section?
In a virtual machine level 1 would represent the computer's digital logic hardware. Level 2 is the machine code and basic functions like add, multiply, and move show up here. Programs created in machine code can be executed directly on the computers hardware. Assembly language appears at level 3 of the virtual machine concept. Assembly language programs are translated into machine language before being executed on the computer.
- What software utility permits compiled Java programs to run on almost any computer?
Java Virtual Machine is a VM implemented on most computers to execute translated Java Byte Code.
- Name the four virtual machine levels names in this section, from lowest to highest.
Digital logic, Instruction set architecture, Assembly language, High-level language.
- Why don’t programmers write applications in machine language?
It would be very time consuming and difficult. It is all 1's and 0's. No readable syntax
- Machine language is used at which level of the virtual machine shown in Figure 1-1?
Level 2, right above the digital logic / hardware level
- Statements at the assembly language level of a virtual machine are translated into statements at which other level?
They are Translated into machine code at the lower second level to be executed on the computers hardware.
Section 1.3.9 Review
- Explain the term least significant bit (LSB).
Bits are numbered sequentially starting from the right with zero (The least significant bit LSB).
- What is the decimal representation of each of the following unsigned binary integers?
A) 11111000 = 248 B) 11001010 = 202 C) 11110000 = 240
- What is the sum of each pair of binary integers?
A) 00001111 + 00000010 = 17 = 00001001 B) 11010101 + 01101011 = 320 = 101000000 C) 00001111 + 00001111 = 30 = 00011110
- How many bytes are contained in each of the following data types?
A) word 2 bytes (16 bits) B) doubleword 4 bytes (32 bits) C) quadword 8 bytes (64 bits) D) double quadword 16 bytes (128 bits)
- What is the minimum number of binary bits needed to represent each of the following unsigned decimal integers?
A) 65 7 bits B) 409 9 bits C) 16385 15
- What is the hexadecimal representation of each of the following binary numbers?
A) 0011 0101 1101 1010 B) 1100 1110 1010 0011 C) 1111 1110 1101 1011
- What is the binary representation of the following hexadecimal numbers?
A) A4693FBC B) B697C7A1 C) 2B3D9461